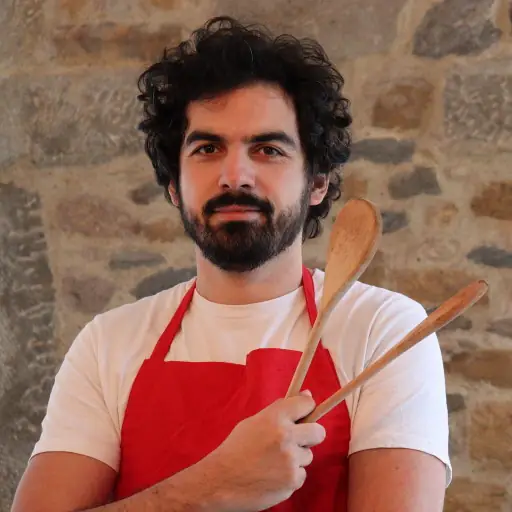
Testing Angular Components Using Cypress
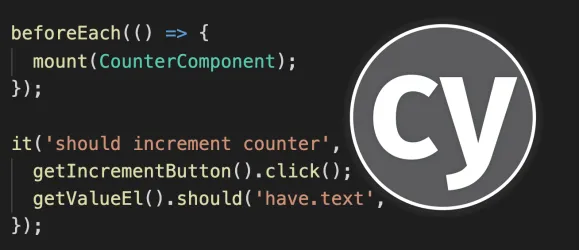
Jest Limitations
<div>
<h1 *ngIf="condition">Marmicode</h1>
</div>
<div *ngIf="condition">
<h1>Marmicode</h1>
</div>
<div>
<h1 [class.hidden]="!condition">Marmicode</h1>
</div>
<div [class.hidden]="!condition">
<h1>Marmicode</h1>
</div>
h1
hidden
div
h1
hidden
h1
Cypress
cy.visit()
Cypress + Storybook Combination Limitations
cy.visit('/iframe.html?id=blogpost--default');
cy.get('h1').contains('Testing Angular Components Using Cypress')
Cypress Component Testing
@jscutlery/cypress-mount
@jscutlery/cypress-mount
import { TitleComponent } from '.../src/title.component';
it('should display default title', () => {
mount(TitleComponent);
cy.get('h1').contains('👋 Welcome');
});
@jscutlery/cypress-mount
@jscutlery/cypress-mount
Control Inputs
mount(TitleComponent, {
inputs: {appName: 'Marmicode'}
});
cy.get('h1').contains('👋 Welcome to Marmicode');
Override Providers
mount(TitleComponent, {
providers: [
{
provide: Settings,
useValue: {greetings: '🇫🇷 Bienvenue'}
}
]
});
cy.get('h1').contains('🇫🇷 Bienvenue');
Mount Template
mount(`<mc-title></mc-title>`, {
imports: [TitleModule],
})
Storybook & Component Story Format support
@jscutlery/cypress-mount
import { Love } from '.../love.stories.ts';
describe('Love', () => {
it('should show some love', () => {
mountStory(Love);
cy.get('h1').contains('❤️');
});
});
Try it yourself
git clone https://github.com/jscutlery/test-utils
npm install
npx nx e2e cypress-mount-integration --watch